2.3 Running your first R Code
Enough of the boring stuff, let’s run some R code! Normally you will run R on your computer, but since you may not have R installed yet, let’s run some R code using a website first. As you run code, you’ll see some of the things R can do. In a browser, navigate to rdrr.io/snippets, where you’ll see a box that looks like this:
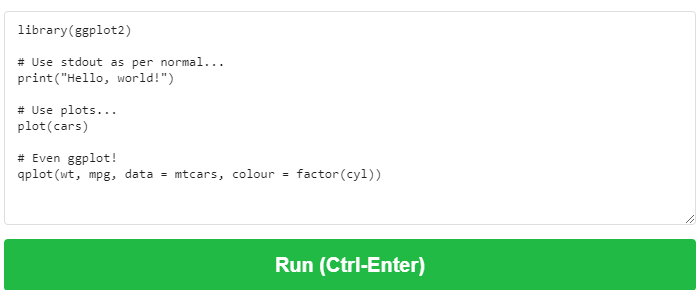
Figure 2.2: rdrr code entry box
The box comes with some code entered already, but we want to use our own code instead, so delete all the text, from before library(ggplot2)
to after factor(cyl))
.
In its place, type 1+1
, then click the big green “Run” button.
You should see the [1] 2
displayed below.
So if you give R a math expression, it will evaluate it and give the result.
Note: the “correct answer” to \(1+1\) is 2
, but the output also displays [1]
, which we won’t explain until later, so you can ignore that for now.
Next, delete the code you just wrote and type (or copy/paste) the following, and run it:
The result should be a very large number, which is equivalent to \(10!\), that is, \(10\times9\times8\times7\times6\times5\times4\times3\times2\times1\). This is an example of an R function, which we will discuss more later.
Aside from math, R can produce plots. Try copy/pasting the following code into the website:
You should see points in a scatter plot which follow a parabola. Here’s a more complicated example, which you should copy/paste into the website and run:
library(ggplot2)
theme_set(theme_bw())
ggplot(mtcars, aes(y = mpg, fill = as.factor(cyl))) +
geom_boxplot() +
labs(title = "Engine Fuel Efficiency vs. Number of Cylinders",
y = "MPG",
fill = "Cylinders") +
theme(legend.position = "bottom",
axis.ticks.x = element_blank(),
axis.text.x = element_blank())
R can be used to make many types of visualizations, which you will do more of later.
This may be the first time you’ve seen R, so it’s okay if you don’t understand how to read this code. We’ll talk more later about what each statement is doing, but for now, here is a brief description of some of the code above:
-
-10:10
This creates a sequence of numbers starting from -10 and ending at 10. That is, \(-10, -9, -8, \ldots, 8, 9, 10\). -
library
This is a function which loads an R package. R packages provide extra abilities to R.
Any feedback for this section? Click here